Why Rust and Bevy are awesome.
I recently stumbled upon Bevy, "a refreshingly simple data-driven game engine built in Rust" and started making a simple game with it to check it out. Bevy is built with Rust and uses ECS so it should be fast, probably much faster than even Unity's ECS. While I didn't finish I am totally sold on Bevy and Rust. They are awesome and I can't wait to see Bevy mature and grow.
What is Rust and why is it awesome?
People like Rust
According to Stack Overflow's developer survey Rust has been the most loved programming language since 2016. I made sure to include C# which Unity uses. C and C++ were so far down I had to cut them off.
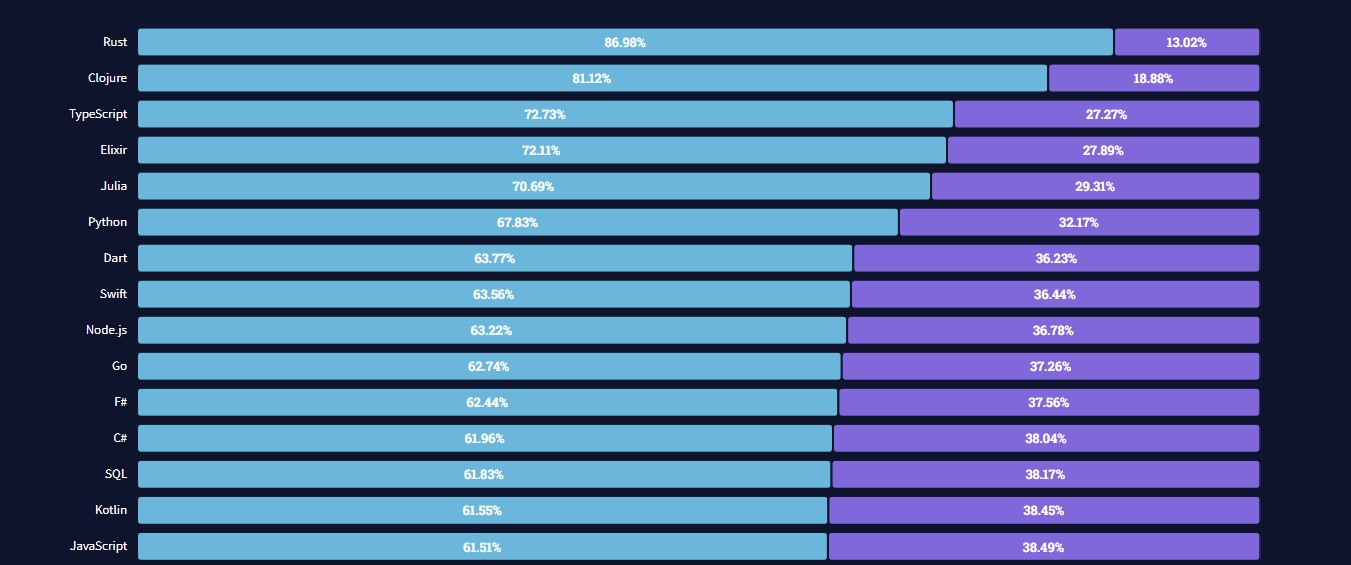
Rust is FAST
Rust is about as fast as C and C++ and maybe twice as fast as C# in this test.
.svg.png)
Rust doesn't crash
One of Rust's main benefits is being memory safe. About 70% of the bugs in Microsft products and Chrome are because of memory errors. Here's an example of one.
int main()
{
char str1[20];
printf("Enter name: ");
scanf("%s", str1);
return(0);
}
This C code will blow up if someone enters more than 20 characters. There's no warning or error when you write it and it compiles fine. With Rust however this would not only give you an error it would probably suggest how to fix it.
Another Rust feature is safe concurrency. Here's an example in C that runs with no crash but the result will be... who knows.
int x = 0, y = 0;
void thread1() {
x = 1;
if (0 == y) x = 2;
}
void thread2() {
y = 1;
if (0 == x) y = 2;
}
Rust fixes this problem because of the way it handles ownership and it's borrow checker. Concurrency is much easier in Rust.
There's more
Not only is it possible to write a Rust program that is guarenteed to not leak memory or crash but Rust also has great tools built around it. For example it's errors messages not only tell you what's wrong but in a lot of cases teaches you how to fix it and can even fix it for you.
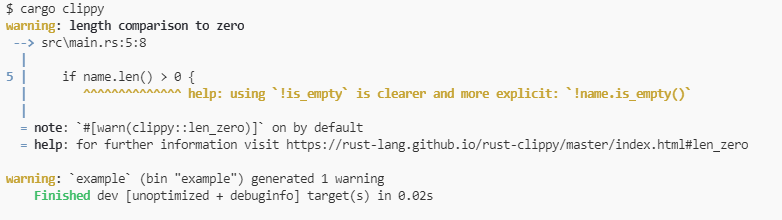
Other features include a package manager similar to Javascript but better. It can run on just about anything even in browsers faster than Javascript by compiling to WebAssembly.
Some links to learn more about Rust.